Vigenère cipher in Ruby Announcing the arrival of Valued Associate #679: Cesar Manara Unicorn Meta Zoo #1: Why another podcast?Encrypter - Double Vigenere Cipher in PythonVigenère cipher in CVigenère cipher 2Vigenère Cipher in HaskellSimple Caesar cipher cryptanalysis in RubyVigenere cipher with random saltVigenère cipher in PythonBrute-force Vigenere Cipher using multiple threadsVigenere Cipher in CCracking Vigenere and Caesar Ciphered Text in Python
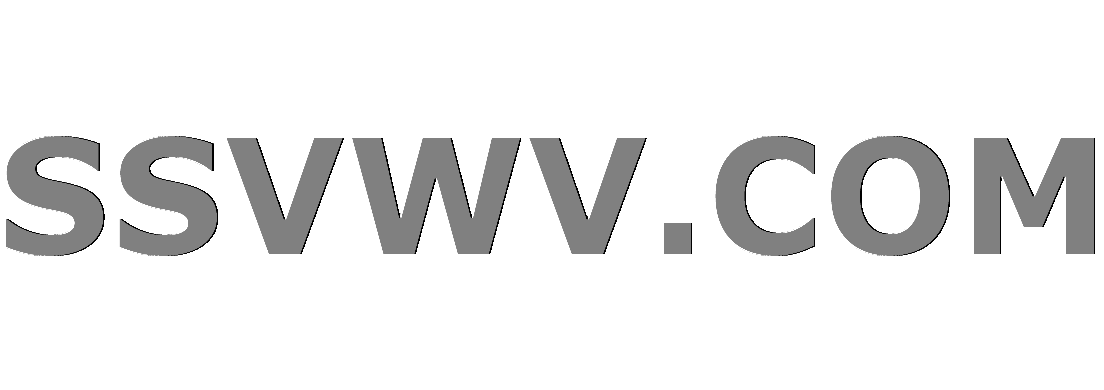
Multi tool use
What is /etc/mtab in Linux?
Prove the alternating sum of a decreasing sequence converging to 0 is Cauchy.
Is a 5 watt UHF/VHF handheld considered QRP?
How do I check if a string is entirely made of the same substring?
Would reducing the reference voltage of an ADC have any effect on accuracy?
How to open locks without disable device?
Do I need to protect SFP ports and optics from dust/contaminants? If so, how?
What is it called when you ride around on your front wheel?
"Whatever a Russian does, they end up making the Kalashnikov gun"? Are there any similar proverbs in English?
A strange hotel
How would this chord from "Rocket Man" be analyzed?
What’s with the clanks in Endgame?
What ability score does a Hexblade's Pact Weapon use for attack and damage when wielded by another character?
What is the term for a person whose job is to place products on shelves in stores?
Is Electric Central Heating worth it if using Solar Panels?
Putting Ant-Man on house arrest
How to translate "red flag" into Spanish?
Mistake in years of experience in resume?
What is the least dense liquid under normal conditions?
How to use @AuraEnabled base class method in Lightning Component?
Multiple options vs single option UI
Does Mathematica have an implementation of the Poisson binomial distribution?
Check if a string is entirely made of the same substring
How long after the last departure shall the airport stay open for an emergency return?
Vigenère cipher in Ruby
Announcing the arrival of Valued Associate #679: Cesar Manara
Unicorn Meta Zoo #1: Why another podcast?Encrypter - Double Vigenere Cipher in PythonVigenère cipher in CVigenère cipher 2Vigenère Cipher in HaskellSimple Caesar cipher cryptanalysis in RubyVigenere cipher with random saltVigenère cipher in PythonBrute-force Vigenere Cipher using multiple threadsVigenere Cipher in CCracking Vigenere and Caesar Ciphered Text in Python
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty margin-bottom:0;
$begingroup$
What I'm trying to do: implement the Vigenère cipher in Ruby. I already have a working version, but I want to make sure it is efficient and well-designed.
module Crypto
# Vigenère cipher encryption and decryption abstraction
module Vigenere
LETTERS = ('a'..'z').to_a.freeze
private_constant :LETTERS
module_function
# Encrypts a string
#
# @param string [String] the string that will be encrypted
# @param key [String] the key that will be used to encrypt the string
#
# @return [String] the encrypted string
def encrypt(string:, key:)
key = make_key(length: string.length, key: key)
string.length.times.map i.join
end
# Decrypts an encrypted string
#
# @param string [String] the encrypted string that will be decrypted
# @param key [String] the key that will be used to decrypt the string
#
# @return [String] the decrypted string
def decrypt(string:, key:)
key = make_key(length: string.length, key: key)
string.length.times.map .join
end
# Repeats a word until it matches a certain length
#
# @param length [Integer] the length of the word being encrypted/decrypted
# @param key [String] the word that will be repeated
#
# @return [String] the word in its new form
def make_key(length:, key:)
i = 0
length.times do
i = 0 if i == key.length
break if key.length == length
key << key[i]
i += 1
end
key
end
private_class_method :make_key
end
end
I do have some specific questions:
1. private_class_method
This is the best way I found to define a private method in a module, but it feels weird to me. Isn't there a better way to do that? My first implementation was this:
module Crypto
class Vigenere
class << self
def encrypt # ...
def decrypt # ...
private
def make_key # ...
end
end
end
which was fine for me. But then I read this rule on the Ruby Style Guide repository. So I switched to using module
, but it doesn't feel right to use private methods in this structure. Am I wrong?
2. reseting a counter (index)
Take a look at this snippet of code:
def make_key(length:, key:)
i = 0
length.times do
i = 0 if i == key.length
break if key.length == length
key << key[i]
i += 1
end
key
end
Defining a counter (i
) and manually incrementing it... looks awkward, doesn't it (at least in the Ruby world)? Is there a better way to do this?
3. valid multi-line block with curly braces?
Now take a look at this snippet:
string.length.times.map i.join
I know most Ruby developers tend to use curly braces only for one-line blocks and do-end
for multi-line blocks, but this time it seems okay using curly braces with a multi-line block, since I'm chaining #join
right after. What would you do:
1. use do-end, store it in a variable and invoke #join after that
new_letters = string.length.times.map do |i|
p = LETTERS.find_index(string[i])
k = LETTERS.find_index(key[i])
LETTERS[(p + k) % 26]
end
new_letters.join
2. what I did (use curly braces even with multi-line block and chain #join)
And of course, if you have any other observations, please share.
ruby vigenere-cipher
New contributor
sPaGhEtTiCaSe is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
add a comment |
$begingroup$
What I'm trying to do: implement the Vigenère cipher in Ruby. I already have a working version, but I want to make sure it is efficient and well-designed.
module Crypto
# Vigenère cipher encryption and decryption abstraction
module Vigenere
LETTERS = ('a'..'z').to_a.freeze
private_constant :LETTERS
module_function
# Encrypts a string
#
# @param string [String] the string that will be encrypted
# @param key [String] the key that will be used to encrypt the string
#
# @return [String] the encrypted string
def encrypt(string:, key:)
key = make_key(length: string.length, key: key)
string.length.times.map i.join
end
# Decrypts an encrypted string
#
# @param string [String] the encrypted string that will be decrypted
# @param key [String] the key that will be used to decrypt the string
#
# @return [String] the decrypted string
def decrypt(string:, key:)
key = make_key(length: string.length, key: key)
string.length.times.map .join
end
# Repeats a word until it matches a certain length
#
# @param length [Integer] the length of the word being encrypted/decrypted
# @param key [String] the word that will be repeated
#
# @return [String] the word in its new form
def make_key(length:, key:)
i = 0
length.times do
i = 0 if i == key.length
break if key.length == length
key << key[i]
i += 1
end
key
end
private_class_method :make_key
end
end
I do have some specific questions:
1. private_class_method
This is the best way I found to define a private method in a module, but it feels weird to me. Isn't there a better way to do that? My first implementation was this:
module Crypto
class Vigenere
class << self
def encrypt # ...
def decrypt # ...
private
def make_key # ...
end
end
end
which was fine for me. But then I read this rule on the Ruby Style Guide repository. So I switched to using module
, but it doesn't feel right to use private methods in this structure. Am I wrong?
2. reseting a counter (index)
Take a look at this snippet of code:
def make_key(length:, key:)
i = 0
length.times do
i = 0 if i == key.length
break if key.length == length
key << key[i]
i += 1
end
key
end
Defining a counter (i
) and manually incrementing it... looks awkward, doesn't it (at least in the Ruby world)? Is there a better way to do this?
3. valid multi-line block with curly braces?
Now take a look at this snippet:
string.length.times.map i.join
I know most Ruby developers tend to use curly braces only for one-line blocks and do-end
for multi-line blocks, but this time it seems okay using curly braces with a multi-line block, since I'm chaining #join
right after. What would you do:
1. use do-end, store it in a variable and invoke #join after that
new_letters = string.length.times.map do |i|
p = LETTERS.find_index(string[i])
k = LETTERS.find_index(key[i])
LETTERS[(p + k) % 26]
end
new_letters.join
2. what I did (use curly braces even with multi-line block and chain #join)
And of course, if you have any other observations, please share.
ruby vigenere-cipher
New contributor
sPaGhEtTiCaSe is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
1
$begingroup$
If you take the time to spell Vigenère correctly then that's enough for me to tell that the code is going to be all right :)
$endgroup$
– Maarten Bodewes
4 hours ago
add a comment |
$begingroup$
What I'm trying to do: implement the Vigenère cipher in Ruby. I already have a working version, but I want to make sure it is efficient and well-designed.
module Crypto
# Vigenère cipher encryption and decryption abstraction
module Vigenere
LETTERS = ('a'..'z').to_a.freeze
private_constant :LETTERS
module_function
# Encrypts a string
#
# @param string [String] the string that will be encrypted
# @param key [String] the key that will be used to encrypt the string
#
# @return [String] the encrypted string
def encrypt(string:, key:)
key = make_key(length: string.length, key: key)
string.length.times.map i.join
end
# Decrypts an encrypted string
#
# @param string [String] the encrypted string that will be decrypted
# @param key [String] the key that will be used to decrypt the string
#
# @return [String] the decrypted string
def decrypt(string:, key:)
key = make_key(length: string.length, key: key)
string.length.times.map .join
end
# Repeats a word until it matches a certain length
#
# @param length [Integer] the length of the word being encrypted/decrypted
# @param key [String] the word that will be repeated
#
# @return [String] the word in its new form
def make_key(length:, key:)
i = 0
length.times do
i = 0 if i == key.length
break if key.length == length
key << key[i]
i += 1
end
key
end
private_class_method :make_key
end
end
I do have some specific questions:
1. private_class_method
This is the best way I found to define a private method in a module, but it feels weird to me. Isn't there a better way to do that? My first implementation was this:
module Crypto
class Vigenere
class << self
def encrypt # ...
def decrypt # ...
private
def make_key # ...
end
end
end
which was fine for me. But then I read this rule on the Ruby Style Guide repository. So I switched to using module
, but it doesn't feel right to use private methods in this structure. Am I wrong?
2. reseting a counter (index)
Take a look at this snippet of code:
def make_key(length:, key:)
i = 0
length.times do
i = 0 if i == key.length
break if key.length == length
key << key[i]
i += 1
end
key
end
Defining a counter (i
) and manually incrementing it... looks awkward, doesn't it (at least in the Ruby world)? Is there a better way to do this?
3. valid multi-line block with curly braces?
Now take a look at this snippet:
string.length.times.map i.join
I know most Ruby developers tend to use curly braces only for one-line blocks and do-end
for multi-line blocks, but this time it seems okay using curly braces with a multi-line block, since I'm chaining #join
right after. What would you do:
1. use do-end, store it in a variable and invoke #join after that
new_letters = string.length.times.map do |i|
p = LETTERS.find_index(string[i])
k = LETTERS.find_index(key[i])
LETTERS[(p + k) % 26]
end
new_letters.join
2. what I did (use curly braces even with multi-line block and chain #join)
And of course, if you have any other observations, please share.
ruby vigenere-cipher
New contributor
sPaGhEtTiCaSe is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
$endgroup$
What I'm trying to do: implement the Vigenère cipher in Ruby. I already have a working version, but I want to make sure it is efficient and well-designed.
module Crypto
# Vigenère cipher encryption and decryption abstraction
module Vigenere
LETTERS = ('a'..'z').to_a.freeze
private_constant :LETTERS
module_function
# Encrypts a string
#
# @param string [String] the string that will be encrypted
# @param key [String] the key that will be used to encrypt the string
#
# @return [String] the encrypted string
def encrypt(string:, key:)
key = make_key(length: string.length, key: key)
string.length.times.map i.join
end
# Decrypts an encrypted string
#
# @param string [String] the encrypted string that will be decrypted
# @param key [String] the key that will be used to decrypt the string
#
# @return [String] the decrypted string
def decrypt(string:, key:)
key = make_key(length: string.length, key: key)
string.length.times.map .join
end
# Repeats a word until it matches a certain length
#
# @param length [Integer] the length of the word being encrypted/decrypted
# @param key [String] the word that will be repeated
#
# @return [String] the word in its new form
def make_key(length:, key:)
i = 0
length.times do
i = 0 if i == key.length
break if key.length == length
key << key[i]
i += 1
end
key
end
private_class_method :make_key
end
end
I do have some specific questions:
1. private_class_method
This is the best way I found to define a private method in a module, but it feels weird to me. Isn't there a better way to do that? My first implementation was this:
module Crypto
class Vigenere
class << self
def encrypt # ...
def decrypt # ...
private
def make_key # ...
end
end
end
which was fine for me. But then I read this rule on the Ruby Style Guide repository. So I switched to using module
, but it doesn't feel right to use private methods in this structure. Am I wrong?
2. reseting a counter (index)
Take a look at this snippet of code:
def make_key(length:, key:)
i = 0
length.times do
i = 0 if i == key.length
break if key.length == length
key << key[i]
i += 1
end
key
end
Defining a counter (i
) and manually incrementing it... looks awkward, doesn't it (at least in the Ruby world)? Is there a better way to do this?
3. valid multi-line block with curly braces?
Now take a look at this snippet:
string.length.times.map i.join
I know most Ruby developers tend to use curly braces only for one-line blocks and do-end
for multi-line blocks, but this time it seems okay using curly braces with a multi-line block, since I'm chaining #join
right after. What would you do:
1. use do-end, store it in a variable and invoke #join after that
new_letters = string.length.times.map do |i|
p = LETTERS.find_index(string[i])
k = LETTERS.find_index(key[i])
LETTERS[(p + k) % 26]
end
new_letters.join
2. what I did (use curly braces even with multi-line block and chain #join)
And of course, if you have any other observations, please share.
ruby vigenere-cipher
ruby vigenere-cipher
New contributor
sPaGhEtTiCaSe is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
sPaGhEtTiCaSe is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
sPaGhEtTiCaSe is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 5 hours ago


sPaGhEtTiCaSesPaGhEtTiCaSe
183
183
New contributor
sPaGhEtTiCaSe is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
sPaGhEtTiCaSe is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
sPaGhEtTiCaSe is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
1
$begingroup$
If you take the time to spell Vigenère correctly then that's enough for me to tell that the code is going to be all right :)
$endgroup$
– Maarten Bodewes
4 hours ago
add a comment |
1
$begingroup$
If you take the time to spell Vigenère correctly then that's enough for me to tell that the code is going to be all right :)
$endgroup$
– Maarten Bodewes
4 hours ago
1
1
$begingroup$
If you take the time to spell Vigenère correctly then that's enough for me to tell that the code is going to be all right :)
$endgroup$
– Maarten Bodewes
4 hours ago
$begingroup$
If you take the time to spell Vigenère correctly then that's enough for me to tell that the code is going to be all right :)
$endgroup$
– Maarten Bodewes
4 hours ago
add a comment |
2 Answers
2
active
oldest
votes
$begingroup$
The code is rather clear, so consider the following to be nitpicks.
I'd say that you are generating a new key stream from the key. I'd certainly not reuse the key
variable.
The first i = 0
before the loop seems spurious.
Using i
as a counter is well understood, and I'd not worry overly much on the style of it. You are probably the only one who cares if it is really Ruby-esk; developers down the line will understand it.
What I wonder though is that you run your loop length
times, but there is a break
that seems to trigger before that. That's not all too clear to me.
I wonder what happens if you supply it an "empty" key string. Some guard statements may be in order.
Same for the curly braces. It's clear as it is, choose whatever you want. Personally I slightly favor the braces.
You could consider creating a mod
function, however since %
is already the modulus, which will never return a negative value if the right value is positive, it seems to me that removing the + 26
is probably the only thing you need to change (during decryption).
Instead of using 26 as unexplained magic value, you should get the size of the LETTERS
range instead. That way you can also expand your ciphertext later.
I've got no opinion on the private_class_method
as I'm not a Ruby developer (I'm specialized in knowing many languages / constructs and of course applied crypto).
$endgroup$
$begingroup$
Thanks, that's really helpful! Sadly, I don't have enough reputation to upvote your answer. :(
$endgroup$
– sPaGhEtTiCaSe
2 hours ago
add a comment |
$begingroup$
Looping using string.length.times.map i
and length.times do …
is OK, but slightly on the awkward side.
To extend the key, you can use the string multiplication operator. (Note that extending the key longer than necessary doesn't do much harm.)
You can also factor out more of the commonality between the encrypt
and decrypt
functions.
Instead of searching the LETTERS
array, I recommend performing arithmetic on ASCII codes.
module Crypto
module Vigenere
module_function
def encrypt(plaintext, key)
vigenere(plaintext, key) p, k
end
def decrypt(ciphertext, key)
vigenere(ciphertext, key) (c - k + 26) % 26
end
def vigenere(text, key, &combiner)
a = 'a'.ord
ext_key = key * (text.length / key.length + 1)
text.chars.zip(ext_key.chars).collect do |t, k|
(a + combiner.call(t.ord - a, k.ord - a)).chr
end.join
end
private_class_method :vigenere
end
end
$endgroup$
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "196"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
sPaGhEtTiCaSe is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f219053%2fvigen%25c3%25a8re-cipher-in-ruby%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
The code is rather clear, so consider the following to be nitpicks.
I'd say that you are generating a new key stream from the key. I'd certainly not reuse the key
variable.
The first i = 0
before the loop seems spurious.
Using i
as a counter is well understood, and I'd not worry overly much on the style of it. You are probably the only one who cares if it is really Ruby-esk; developers down the line will understand it.
What I wonder though is that you run your loop length
times, but there is a break
that seems to trigger before that. That's not all too clear to me.
I wonder what happens if you supply it an "empty" key string. Some guard statements may be in order.
Same for the curly braces. It's clear as it is, choose whatever you want. Personally I slightly favor the braces.
You could consider creating a mod
function, however since %
is already the modulus, which will never return a negative value if the right value is positive, it seems to me that removing the + 26
is probably the only thing you need to change (during decryption).
Instead of using 26 as unexplained magic value, you should get the size of the LETTERS
range instead. That way you can also expand your ciphertext later.
I've got no opinion on the private_class_method
as I'm not a Ruby developer (I'm specialized in knowing many languages / constructs and of course applied crypto).
$endgroup$
$begingroup$
Thanks, that's really helpful! Sadly, I don't have enough reputation to upvote your answer. :(
$endgroup$
– sPaGhEtTiCaSe
2 hours ago
add a comment |
$begingroup$
The code is rather clear, so consider the following to be nitpicks.
I'd say that you are generating a new key stream from the key. I'd certainly not reuse the key
variable.
The first i = 0
before the loop seems spurious.
Using i
as a counter is well understood, and I'd not worry overly much on the style of it. You are probably the only one who cares if it is really Ruby-esk; developers down the line will understand it.
What I wonder though is that you run your loop length
times, but there is a break
that seems to trigger before that. That's not all too clear to me.
I wonder what happens if you supply it an "empty" key string. Some guard statements may be in order.
Same for the curly braces. It's clear as it is, choose whatever you want. Personally I slightly favor the braces.
You could consider creating a mod
function, however since %
is already the modulus, which will never return a negative value if the right value is positive, it seems to me that removing the + 26
is probably the only thing you need to change (during decryption).
Instead of using 26 as unexplained magic value, you should get the size of the LETTERS
range instead. That way you can also expand your ciphertext later.
I've got no opinion on the private_class_method
as I'm not a Ruby developer (I'm specialized in knowing many languages / constructs and of course applied crypto).
$endgroup$
$begingroup$
Thanks, that's really helpful! Sadly, I don't have enough reputation to upvote your answer. :(
$endgroup$
– sPaGhEtTiCaSe
2 hours ago
add a comment |
$begingroup$
The code is rather clear, so consider the following to be nitpicks.
I'd say that you are generating a new key stream from the key. I'd certainly not reuse the key
variable.
The first i = 0
before the loop seems spurious.
Using i
as a counter is well understood, and I'd not worry overly much on the style of it. You are probably the only one who cares if it is really Ruby-esk; developers down the line will understand it.
What I wonder though is that you run your loop length
times, but there is a break
that seems to trigger before that. That's not all too clear to me.
I wonder what happens if you supply it an "empty" key string. Some guard statements may be in order.
Same for the curly braces. It's clear as it is, choose whatever you want. Personally I slightly favor the braces.
You could consider creating a mod
function, however since %
is already the modulus, which will never return a negative value if the right value is positive, it seems to me that removing the + 26
is probably the only thing you need to change (during decryption).
Instead of using 26 as unexplained magic value, you should get the size of the LETTERS
range instead. That way you can also expand your ciphertext later.
I've got no opinion on the private_class_method
as I'm not a Ruby developer (I'm specialized in knowing many languages / constructs and of course applied crypto).
$endgroup$
The code is rather clear, so consider the following to be nitpicks.
I'd say that you are generating a new key stream from the key. I'd certainly not reuse the key
variable.
The first i = 0
before the loop seems spurious.
Using i
as a counter is well understood, and I'd not worry overly much on the style of it. You are probably the only one who cares if it is really Ruby-esk; developers down the line will understand it.
What I wonder though is that you run your loop length
times, but there is a break
that seems to trigger before that. That's not all too clear to me.
I wonder what happens if you supply it an "empty" key string. Some guard statements may be in order.
Same for the curly braces. It's clear as it is, choose whatever you want. Personally I slightly favor the braces.
You could consider creating a mod
function, however since %
is already the modulus, which will never return a negative value if the right value is positive, it seems to me that removing the + 26
is probably the only thing you need to change (during decryption).
Instead of using 26 as unexplained magic value, you should get the size of the LETTERS
range instead. That way you can also expand your ciphertext later.
I've got no opinion on the private_class_method
as I'm not a Ruby developer (I'm specialized in knowing many languages / constructs and of course applied crypto).
edited 3 hours ago
answered 4 hours ago


Maarten BodewesMaarten Bodewes
582212
582212
$begingroup$
Thanks, that's really helpful! Sadly, I don't have enough reputation to upvote your answer. :(
$endgroup$
– sPaGhEtTiCaSe
2 hours ago
add a comment |
$begingroup$
Thanks, that's really helpful! Sadly, I don't have enough reputation to upvote your answer. :(
$endgroup$
– sPaGhEtTiCaSe
2 hours ago
$begingroup$
Thanks, that's really helpful! Sadly, I don't have enough reputation to upvote your answer. :(
$endgroup$
– sPaGhEtTiCaSe
2 hours ago
$begingroup$
Thanks, that's really helpful! Sadly, I don't have enough reputation to upvote your answer. :(
$endgroup$
– sPaGhEtTiCaSe
2 hours ago
add a comment |
$begingroup$
Looping using string.length.times.map i
and length.times do …
is OK, but slightly on the awkward side.
To extend the key, you can use the string multiplication operator. (Note that extending the key longer than necessary doesn't do much harm.)
You can also factor out more of the commonality between the encrypt
and decrypt
functions.
Instead of searching the LETTERS
array, I recommend performing arithmetic on ASCII codes.
module Crypto
module Vigenere
module_function
def encrypt(plaintext, key)
vigenere(plaintext, key) p, k
end
def decrypt(ciphertext, key)
vigenere(ciphertext, key) (c - k + 26) % 26
end
def vigenere(text, key, &combiner)
a = 'a'.ord
ext_key = key * (text.length / key.length + 1)
text.chars.zip(ext_key.chars).collect do |t, k|
(a + combiner.call(t.ord - a, k.ord - a)).chr
end.join
end
private_class_method :vigenere
end
end
$endgroup$
add a comment |
$begingroup$
Looping using string.length.times.map i
and length.times do …
is OK, but slightly on the awkward side.
To extend the key, you can use the string multiplication operator. (Note that extending the key longer than necessary doesn't do much harm.)
You can also factor out more of the commonality between the encrypt
and decrypt
functions.
Instead of searching the LETTERS
array, I recommend performing arithmetic on ASCII codes.
module Crypto
module Vigenere
module_function
def encrypt(plaintext, key)
vigenere(plaintext, key) p, k
end
def decrypt(ciphertext, key)
vigenere(ciphertext, key) (c - k + 26) % 26
end
def vigenere(text, key, &combiner)
a = 'a'.ord
ext_key = key * (text.length / key.length + 1)
text.chars.zip(ext_key.chars).collect do |t, k|
(a + combiner.call(t.ord - a, k.ord - a)).chr
end.join
end
private_class_method :vigenere
end
end
$endgroup$
add a comment |
$begingroup$
Looping using string.length.times.map i
and length.times do …
is OK, but slightly on the awkward side.
To extend the key, you can use the string multiplication operator. (Note that extending the key longer than necessary doesn't do much harm.)
You can also factor out more of the commonality between the encrypt
and decrypt
functions.
Instead of searching the LETTERS
array, I recommend performing arithmetic on ASCII codes.
module Crypto
module Vigenere
module_function
def encrypt(plaintext, key)
vigenere(plaintext, key) p, k
end
def decrypt(ciphertext, key)
vigenere(ciphertext, key) (c - k + 26) % 26
end
def vigenere(text, key, &combiner)
a = 'a'.ord
ext_key = key * (text.length / key.length + 1)
text.chars.zip(ext_key.chars).collect do |t, k|
(a + combiner.call(t.ord - a, k.ord - a)).chr
end.join
end
private_class_method :vigenere
end
end
$endgroup$
Looping using string.length.times.map i
and length.times do …
is OK, but slightly on the awkward side.
To extend the key, you can use the string multiplication operator. (Note that extending the key longer than necessary doesn't do much harm.)
You can also factor out more of the commonality between the encrypt
and decrypt
functions.
Instead of searching the LETTERS
array, I recommend performing arithmetic on ASCII codes.
module Crypto
module Vigenere
module_function
def encrypt(plaintext, key)
vigenere(plaintext, key) p, k
end
def decrypt(ciphertext, key)
vigenere(ciphertext, key) (c - k + 26) % 26
end
def vigenere(text, key, &combiner)
a = 'a'.ord
ext_key = key * (text.length / key.length + 1)
text.chars.zip(ext_key.chars).collect do |t, k|
(a + combiner.call(t.ord - a, k.ord - a)).chr
end.join
end
private_class_method :vigenere
end
end
answered 33 mins ago


200_success200_success
131k17157422
131k17157422
add a comment |
add a comment |
sPaGhEtTiCaSe is a new contributor. Be nice, and check out our Code of Conduct.
sPaGhEtTiCaSe is a new contributor. Be nice, and check out our Code of Conduct.
sPaGhEtTiCaSe is a new contributor. Be nice, and check out our Code of Conduct.
sPaGhEtTiCaSe is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f219053%2fvigen%25c3%25a8re-cipher-in-ruby%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
2ty,K,pUcsW u TOIklX7trQVtwcrCoWR7Y,eMuxP f
1
$begingroup$
If you take the time to spell Vigenère correctly then that's enough for me to tell that the code is going to be all right :)
$endgroup$
– Maarten Bodewes
4 hours ago